Enhancing Spring-Based Applications with HD Compression
Introduction
With the increasing demand for high-definition (HD) video content, there is a growing need to optimize the delivery of these resources over the internet. Spring, a popular Java-based web application framework, provides numerous features to build robust and scalable applications. However, when it comes to handling HD video content, traditional approaches may not be sufficient. In this article, we will explore how to enhance Spring-based applications with HD compression to improve performance, reduce bandwidth usage, and enhance user experience.
HD Video Compression in Spring
Compression is a crucial aspect of optimizing HD video delivery. By reducing the size of video files, we can significantly decrease the time it takes to download or stream them, leading to faster load times and improved overall performance. There are several compression algorithms available, but one of the most popular is the H.264 codec, which offers high compression ratios without significant loss of quality.
To incorporate HD compression in a Spring-based application, we can use various libraries and tools that support H.264 encoding and decoding. One such library is Xuggler, which provides a Java API for manipulating multimedia content. Xuggler allows us to easily encode and decode video streams, as well as perform various operations like cropping, scaling, and rotating.
Implementing HD Compression in Spring
To implement HD compression in a Spring-based application, we can follow these steps
1. Add the necessary dependencies to your project's pom.xml file or build.gradle file, depending on your build system. For example, to use Xuggler, you would need to add the following dependencies
```xml
com.xuggle
xuggle-core
5.4
com.xuggle
xuggle-javafx
5.4
```
1. Create a service class that will handle the video compression logic. This class should have methods for encoding and decoding video streams using Xuggler. Here's an example implementation
```java
@Service
public class VideoCompressionService {
private static final Logger logger = LoggerFactory.getLogger(VideoCompressionService.class);
public byte[] compressVideo(byte[] inputVideo, int width, int height, double compressionQuality) throws IOException {
IConverter converter = ConverterFactory.createConverter(IConverter.Type.CODEC);
converter.setInputFormat(png, width, height);
converter.setOutputFormat(mp4v, width, height);
converter.setOutputFormat(mp4, width, height);
IStreamCoder coder = converter.getStreamCoder();
coder.setBitRate(width * height * 384);
coder.setCodecID(ICodec.ID.CODEC_ID_H264);
coder.setQuality(compressionQuality);
IBufferFormat format = IBufferFormat.createAudioSampleFormat(audio, audio/mp4a-latm, 1, 44100, null, 2, null, null, 0);
coder.setSampleFormat(format);
coder.setTimeBase(new Rational(1, 25));
codersetTimeBase(new Rational(1, 25));
coder
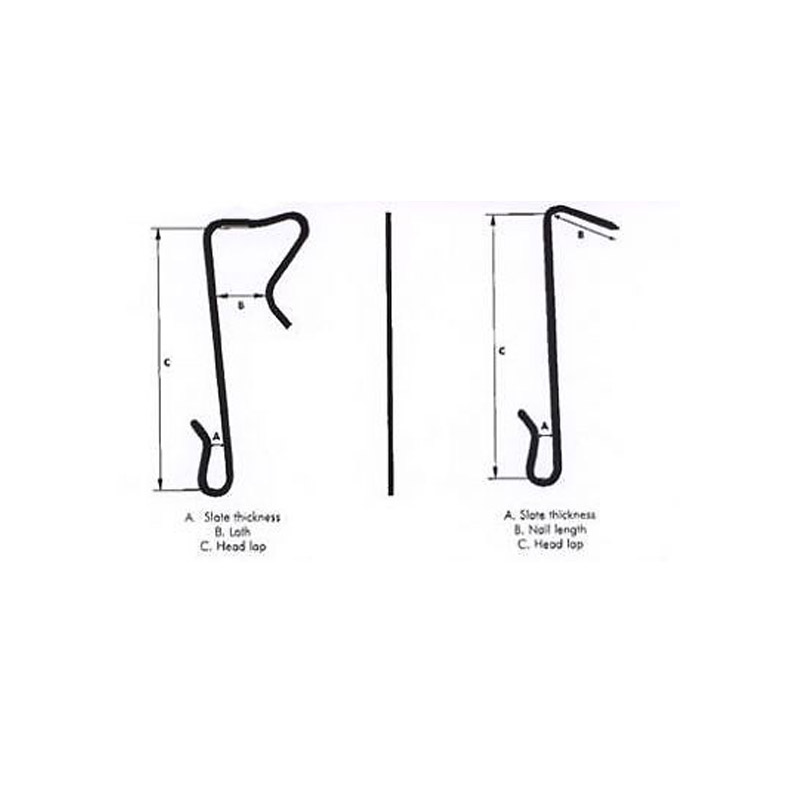
setTimeBase(new Rational(1, 25));
codersetTimeBase(new Rational(1, 25));
coder
hd compression spring.open();
IBuffer buffer = IBuffer.allocate(inputVideo.length);
buffer.setData(inputVideo);
buffer.setSize(inputVideo.length);
while (buffer.getData() != null && !coder.isKeyFrame()) {
buffer = coder.encodeBuffer(buffer);
}
buffer = coder.encodeBuffer(buffer);
coder.close();
return converter.getOutput();
}
public byte[] decompressVideo(byte[] compressedVideo) throws IOException {
IConverter converter = ConverterFactory.createConverter(IConverter.Type.CODEC);
converter.setInputFormat(mp4, 1920, 1080);
converter.setOutputFormat(png, 1920, 1080);
IStreamCoder coder = converter.getStreamCoder();
coder.open();
IBuffer buffer = IBuffer.allocate(compressedVideo.length);
buffer.setData(compressedVideo);
buffer.setSize(compressedVideo.length);
while (buffer.getData() != null) {
buffer = coder.decodeBuffer(buffer);
}
buffer = coder.encodeBuffer(buffer);
coder.close();
return converter.getOutput();
}
}
```
1. In your Spring controller or service class, call the `compressVideo` and `decompressVideo` methods from the `VideoCompressionService` class to handle video compression and decompression respectively.
2. Finally, update your application's configuration to enable GZIP compression for HTTP responses. This can be done by adding the following lines to your application.properties file
```python
server.compression.enabled=true
server.compression.mime-types=video/mp4,application/octet-stream
```
Conclusion
In conclusion, enhancing Spring-based applications with HD compression can significantly improve performance, reduce bandwidth usage, and enhance user experience. By leveraging libraries like Xuggler and enabling GZIP compression for HTTP responses, we can efficiently deliver HD video content to our users, leading to a better overall experience.